Added some sound effects.
jump to
@@ -8,5 +8,9 @@
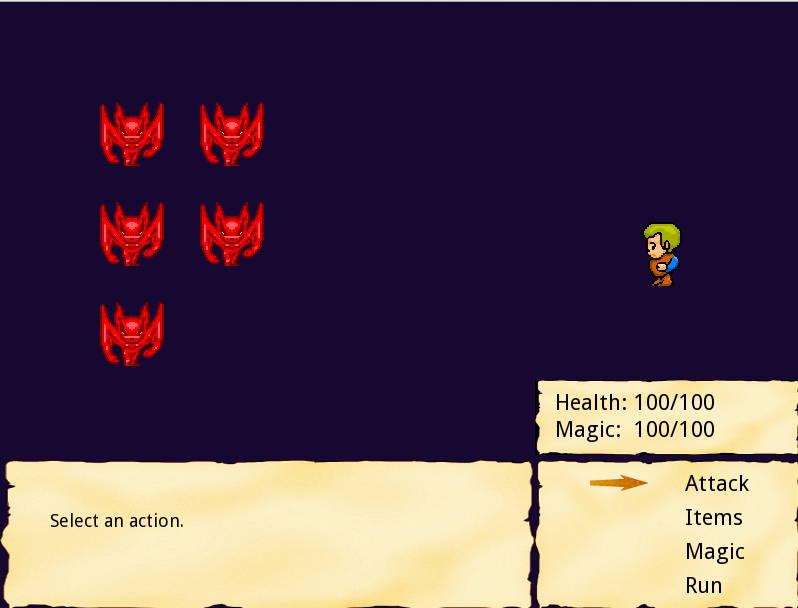 King's Theme by Telaron: opengameart.org/content/the-kings-crowning + Town Theme by Mekathratos: opengameart.org/content/danza-del-bosque + Overworld Theme by bart: opengameart.org/content/adventure-begins + +Shop Theme by Aaron Krogh: https://soundcloud.com/aaron-anderson-11/a-4-coastal-town
@@ -3,7 +3,7 @@ GUI components for battle states.
""" import sys import pygame as pg -from . import setup +from . import setup, observer from . import constants as c #Python 2/3 compatibility.@@ -245,6 +245,15 @@ self.index = 0
self.rect.topleft = self.pos_list[self.index] self.allow_input = False self.enemy_pos_list = enemy_pos_list + self.sound_effect_observer = observer.SoundEffects() + self.observers = [self.sound_effect_observer] + + def notify(self, event): + """ + Notify all observers of events. + """ + for observer in self.observers: + observer.on_notify(event) def make_state_dict(self): """Make state dictionary"""@@ -296,9 +305,11 @@
def check_input(self, keys): if self.allow_input: if keys[pg.K_DOWN] and self.index < (len(self.pos_list) - 1): + self.notify(c.CLICK) self.index += 1 self.allow_input = False elif keys[pg.K_UP] and self.index > 0: + self.notify(c.CLICK) self.index -= 1 self.allow_input = False
@@ -1,7 +1,7 @@
from __future__ import division import math, random, copy import pygame as pg -from .. import setup +from .. import setup, observer from .. import constants as c@@ -36,7 +36,7 @@ self.dialogue = ['Location: ' + str(self.location)]
self.default_direction = direction self.item = None self.wander_box = self.make_wander_box() - self.observers = [] + self.observers = [observer.SoundEffects()] self.health = 0 self.death_image = pg.transform.scale2x(self.image) self.battle = None@@ -299,6 +299,7 @@ def enter_attack_state(self, enemy):
""" Set values for attack state. """ + self.notify(c.SWORD) self.attacked_enemy = enemy self.x_vel = -5 self.state = 'attack'@@ -552,8 +553,6 @@ self.healing = False
self.fade_in = True self.image = self.spritesheet_dict['facing left 2'] self.image = pg.transform.scale2x(self.image) - - def check_for_input(self): """Checks for player input"""
@@ -70,6 +70,11 @@ #EVENTS
END_BATTLE = 'end battle' +#SOUND EFFECTS - +CLICK = 'click' +CLICK2 = 'click2' +CLOTH_BELT = 'cloth_belt' +SWORD = 'sword' +FIRE = 'fire'
@@ -2,6 +2,7 @@ """
Module for all game observers. """ from . import constants as c +from . import setup from .components import attackitems from . import setup@@ -51,6 +52,26 @@ """
Eliminate all traces of enemy. """ self.level.player.attacked_enemy = None + + +class SoundEffects(object): + """ + Observer for sound effects. + """ + def on_notify(self, event): + """ + Observer is notified of SFX event. + """ + if event in setup.SFX: + setup.SFX[event].play() + + + + + + + +
@@ -4,7 +4,7 @@ A Gui object is created and updated by the shop state.
""" import pygame as pg -from . import setup +from . import setup, observer from . components import textbox from . import constants as c@@ -15,6 +15,8 @@ def __init__(self, level):
self.level = level self.game_data = self.level.game_data self.level.game_data['last direction'] = 'down' + self.SFX_observer = observer.SoundEffects() + self.observers = [self.SFX_observer] self.sellable_items = level.sell_items self.player_inventory = level.game_data['player inventory'] self.name = level.name@@ -52,10 +54,17 @@ choices = ['Buy', 'Sell', 'Leave']
self.selection_box = self.make_selection_box(choices) self.state_dict = self.make_state_dict() - + def notify(self, event): + """ + Notify all observers of event. + """ + for observer in self.observers: + observer.on_notify(event) def make_dialogue_box(self, dialogue_list, index): - """Make the sprite that controls the dialogue""" + """ + Make the sprite that controls the dialogue. + """ image = setup.GFX['dialoguebox'] rect = image.get_rect() surface = pg.Surface(rect.size)@@ -73,12 +82,12 @@ self.check_to_draw_arrow(sprite)
return sprite - def check_to_draw_arrow(self, sprite): - """Blink arrow if more text needs to be read""" + """ + Blink arrow if more text needs to be read. + """ if self.index < len(self.dialogue) - 1: sprite.image.blit(self.arrow.image, self.arrow.rect) - def make_gold_box(self): """Make the box to display total gold"""@@ -100,7 +109,6 @@ sprite.image = surface
sprite.rect = rect return sprite - def make_selection_box(self, choices): """Make the box for the player to select options"""@@ -157,7 +165,6 @@ 'cantsellequippedarmor': self.cant_sell_equipped_armor}
return state_dict - def control_dialogue(self, keys, current_time): """Control the dialogue boxes""" self.dialogue_box = self.make_dialogue_box(self.dialogue, self.index)@@ -172,7 +179,6 @@ self.state = self.begin_new_transaction()
if not keys[pg.K_SPACE]: self.allow_input = True - def begin_new_transaction(self): """Set state to buysell or select, depending if the shop@@ -213,10 +219,12 @@ if keys[pg.K_DOWN] and self.allow_input:
if self.arrow_index < (len(choices) - 1): self.arrow_index += 1 self.allow_input = False + self.notify(c.CLICK) elif keys[pg.K_UP] and self.allow_input: if self.arrow_index > 0: self.arrow_index -= 1 self.allow_input = False + self.notify(c.CLICK) elif keys[pg.K_SPACE] and self.allow_input: if self.arrow_index == 0: self.state = 'confirmpurchase'@@ -233,6 +241,7 @@ self.game_data['last state'] = self.level.name
else: self.state = 'buysell' + self.notify(c.CLICK2) self.arrow_index = 0 self.allow_input = False@@ -254,15 +263,18 @@ if keys[pg.K_DOWN] and self.allow_input:
if self.arrow_index < (len(choices) - 1): self.arrow_index += 1 self.allow_input = False + self.notify(c.CLICK) elif keys[pg.K_UP] and self.allow_input: if self.arrow_index > 0: self.arrow_index -= 1 self.allow_input = False + self.notify(c.CLICK) elif keys[pg.K_SPACE] and self.allow_input: if self.arrow_index == 0: self.buy_item() elif self.arrow_index == 1: self.state = self.begin_new_transaction() + self.notify(c.CLICK2) self.arrow_index = 0 self.allow_input = False@@ -285,6 +297,7 @@ not self.name == c.POTION_SHOP):
self.state = 'hasitem' self.player_inventory['GOLD']['quantity'] += item['price'] else: + self.notify(c.CLOTH_BELT) self.state = 'accept' self.add_player_item(item)@@ -329,15 +342,18 @@ if keys[pg.K_DOWN] and self.allow_input:
if self.arrow_index < (len(choices) - 1): self.arrow_index += 1 self.allow_input = False + self.notify(c.CLICK) elif keys[pg.K_UP] and self.allow_input: if self.arrow_index > 0: self.arrow_index -= 1 self.allow_input = False + self.notify(c.CLICK) elif keys[pg.K_SPACE] and self.allow_input: if self.arrow_index == 0: self.sell_item_from_inventory() elif self.arrow_index == 1: self.state = self.begin_new_transaction() + self.notify(c.CLICK2) self.allow_input = False self.arrow_index = 0@@ -356,12 +372,14 @@ if item_name in self.weapon_list:
if item_name == self.game_data['player inventory']['equipped weapon']: self.state = 'cantsellequippedweapon' else: + self.notify(c.CLOTH_BELT) self.sell_inventory_data_adjust(item_price, item_name) elif item_name in self.armor_list: if item_name in self.game_data['player inventory']['equipped armor']: self.state = 'cantsellequippedarmor' else: + self.notify(c.CLOTH_BELT) self.sell_inventory_data_adjust(item_price, item_name) def sell_inventory_data_adjust(self, item_price, item_name):@@ -384,6 +402,7 @@ dialogue = ["You don't have enough gold!"]
self.dialogue_box = self.make_dialogue_box(dialogue, 0) if keys[pg.K_SPACE] and self.allow_input: + self.notify(c.CLICK2) self.state = self.begin_new_transaction() self.selection_arrow.rect.topleft = self.arrow_pos1 self.allow_input = False@@ -398,6 +417,7 @@ self.dialogue_box = self.make_dialogue_box(self.accept_dialogue, 0)
self.gold_box = self.make_gold_box() if keys[pg.K_SPACE] and self.allow_input: + self.notify(c.CLICK2) self.state = self.begin_new_transaction() self.selection_arrow.rect.topleft = self.arrow_pos1 self.allow_input = False@@ -412,6 +432,7 @@ self.dialogue_box = self.make_dialogue_box(self.accept_sale_dialogue, 0)
self.gold_box = self.make_gold_box() if keys[pg.K_SPACE] and self.allow_input: + self.notify(c.CLICK2) self.state = self.begin_new_transaction() self.selection_arrow.rect.topleft = self.arrow_pos1 self.allow_input = False@@ -429,6 +450,7 @@ if keys[pg.K_SPACE] and self.allow_input:
self.state = self.begin_new_transaction() self.selection_arrow.rect.topleft = self.arrow_pos1 self.allow_input = False + self.notify(c.CLICK2) if not keys[pg.K_SPACE]: self.allow_input = True@@ -446,11 +468,13 @@ if keys[pg.K_DOWN] and self.allow_input:
if self.arrow_index < (len(self.arrow_pos_list) - 1): self.arrow_index += 1 self.allow_input = False + self.notify(c.CLICK) elif keys[pg.K_UP] and self.allow_input: if self.arrow_index > 0: self.arrow_index -= 1 self.allow_input = False + self.notify(c.CLICK) elif keys[pg.K_SPACE] and self.allow_input: if self.arrow_index == 0: self.state = 'select'@@ -472,6 +496,7 @@ self.level.done = True
self.game_data['last state'] = self.level.name self.arrow_index = 0 + self.notify(c.CLICK2) if not keys[pg.K_SPACE] and not keys[pg.K_DOWN] and not keys[pg.K_UP]: self.allow_input = True@@ -510,10 +535,12 @@ if keys[pg.K_DOWN] and self.allow_input:
if self.arrow_index < (len(self.arrow_pos_list) - 1): self.arrow_index += 1 self.allow_input = False + self.notify(c.CLICK) elif keys[pg.K_UP] and self.allow_input: if self.arrow_index > 0: self.arrow_index -= 1 self.allow_input = False + self.notify(c.CLICK) elif keys[pg.K_SPACE] and self.allow_input: if self.arrow_index == 0: self.state = 'confirmsell'@@ -532,6 +559,7 @@ else:
self.state = 'buysell' self.allow_input = False self.arrow_index = 0 + self.notify(c.CLICK2) if not keys[pg.K_SPACE] and not keys[pg.K_DOWN] and not keys[pg.K_UP]: self.allow_input = True@@ -545,6 +573,7 @@
if keys[pg.K_SPACE] and self.allow_input: self.state = 'buysell' self.allow_input = False + self.notify(c.CLICK2) if not keys[pg.K_SPACE]:@@ -560,6 +589,7 @@
if keys[pg.K_SPACE] and self.allow_input: self.state = 'buysell' self.allow_input = False + self.notify(c.CLICK2) if not keys[pg.K_SPACE]: self.allow_input = True
@@ -43,6 +43,8 @@
self.select_action_state_dict = self.make_selection_state_dict() self.observers = [observer.Battle(self)] self.player.observers.extend(self.observers) + self.observers.append(observer.SoundEffects()) + print self.player.observers self.damage_points = pg.sprite.Group() self.player_actions = [] self.player_action_dict = self.make_player_action_dict()@@ -418,6 +420,7 @@ def cast_fire_blast(self):
""" Cast fire blast on all enemies. """ + self.notify(c.FIRE) self.state = self.info_box.state = c.FIRE_SPELL POWER = self.inventory['Fire Blast']['power'] MAGIC_POINTS = self.inventory['Fire Blast']['magic points']
@@ -41,12 +41,19 @@ previous, self.state_name = self.state_name, self.state.next
persist = self.state.cleanup() self.state = self.state_dict[self.state_name] self.state.previous = previous + self.state.startup(self.current_time, persist) + self.set_music() + + def set_music(self): + """ + Set music for the new state. + """ if self.state.music: pg.mixer.music.load(self.state.music) + pg.mixer.music.set_volume(.4) pg.mixer.music.play() else: pg.mixer.music.stop() - self.state.startup(self.current_time, persist) def event_loop(self): self.events = pg.event.get()
@@ -0,0 +1,464 @@
+ + + + + + +<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> +<html xmlns:fb="http://www.facebook.com/2008/fbml" xmlns:og="http://ogp.me/ns#" xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en" dir="ltr"> + + <head> + + + <meta http-equiv="Content-type" content="text/html; charset=utf-8" /> + <meta name="description" content="Freesound: collaborative database of creative-commons licensed sound for musicians and sound lovers. Have you freed your sound today?" /> + <meta name="keywords" content="free, sound" /> + <link rel="stylesheet" type="text/css" href="/media/css/all.css?v=2205" /> + <!--[if IE 6]><link rel="stylesheet" type="text/css" href="/media/css/ie6.css?v=2205" /><![endif]--> + <link rel="shortcut icon" href="/media/images/favicon.ico" /> + <title>Freesound.org - "sword-01.wav" by audione</title> + <script src="/media/js/jquery-1.5.2.min.js" type="text/javascript"></script> + <script src="/media/js/jquery-ui-1.8.11.custom.min.js" type="text/javascript"></script> + <script src="/media/js/jquery.cookie.js" type="text/javascript"></script> + <script src="/media/js/swfobject.js" type="text/javascript"></script> + <script src="/media/js/jquery.watermarkinput.js" type="text/javascript"></script> + <script type="text/javascript"> + var isLoggedIn = false; + var loginUrl = "/home/login/?next=/people/audione/sounds/52458/"; + $(document).ready(function() { + $("#search input[name=q]").Watermark("search sounds"); + }); + function clearWatermark(){ + $.Watermark.HideAll() + return true + } + </script> + <script src="/media/js/freesound.js?v=2205" type="text/javascript"></script> + <script src="/media/js/ajax_utils.js" type="text/javascript"></script> + <!-- next couple of lines are used for the HTML player --> + <link href='//fonts.googleapis.com/css?family=Inconsolata&v1' rel='stylesheet' type='text/css' /> + <link rel="stylesheet" type="text/css" href="/media/html_player/player.css" /> + <script src="/media/html_player/soundmanager2.js"></script> + <script type="text/javascript" src="/media/html_player/player.js"></script> + <script type="text/javascript"> + + var _gaq = _gaq || []; + _gaq.push(['_setAccount', 'UA-86270-1']); + _gaq.push(['_trackPageview']); + + (function() { + var ga = document.createElement('script'); ga.type = 'text/javascript'; ga.async = true; + ga.src = ('https:' == document.location.protocol ? 'https://ssl' : 'http://www') + '.google-analytics.com/ga.js'; + var s = document.getElementsByTagName('script')[0]; s.parentNode.insertBefore(ga, s); + })(); + +</script> + + + <script type="text/javascript"> + swfobject.registerObject("player_52458", "10"); + </script> + <meta property="og:title" content="sword-01.wav by audione" /> + <meta property="og:type" content="song" /> + <meta property="og:audio" content="http://www.freesound.org/data/previews/52/52458_631427-lq.mp3" /> + <meta property="og:audio:artist" content="audione" /> + <meta property="og:audio:title" content="sword-01.wav" /> + <meta property="og:url" content="http://www.freesound.org/people/audione/sounds/52458/" /> + <meta property="og:audio:type" content="application/mp3" /> +<!-- <meta property="og:image" content="http://www.freesound.org/data/displays/52/52458_631427_wave_M.png" /> --> + <meta property="og:site_name" content="Freesound.org" /> + <meta property="fb:admins" content="100002130282170" /> + + </head> + + <body id="" onload="" onunload=""> + + + + + <div id="header"> + <div id="account_nav"> + + <a href="/home/register/">Register</a> + <a href="/home/login/?next=/people/audione/sounds/52458/">Log In</a> + + <a href="/home/upload/" id="upload_button"></a> + </div> + + <a href="/" id="logo"></a> + + <div id="search"> + <form method="get" onsubmit="return clearWatermark()" action="/search/"> + <fieldset> + <input type="text" name="q" value="" size="30" /> + <input type="submit" value="" id="search_submit" /> + </fieldset> + </form> + </div> + + <ul id="site_nav"> + + <li class="active_tab"><a href="/browse/" id="site_nav_sounds"><span class="site_nav_icon"></span>Sounds</a></li> + <li class=""><a href="/forum/" id="site_nav_forums"><span class="site_nav_icon"></span>Forums</a></li> + <li class=""><a href="/people/" id="site_nav_people"><span class="site_nav_icon"></span>People</a></li> + <li class=""><a href="/help/faq/" id="site_nav_help"><span class="site_nav_icon"></span>FAQ</a></li> + + </ul> + </div> + + <div id="wrapper"> + <div id="container"> + + + + +<div id="content_full"> <!-- START of Content area --> + + + +<div id="single_sample_header"> + <a href="#">sword-01.wav</a> + <div class="stars"> + <ul class="star-rating"> + <li class="current-rating" style="width:88%;">Currently /5 Stars.</li> + <li><a href="/ratings/add/20/52458/1/" title="pretty bad :-(" class="one-star_not_logged_in">1</a></li> + <li><a href="/ratings/add/20/52458/2/" title="quite good" class="two-stars_not_logged_in">2</a></li> + <li><a href="/ratings/add/20/52458/3/" title="good :-)" class="three-stars_not_logged_in">3</a></li> + <li><a href="/ratings/add/20/52458/4/" title="very good" class="four-stars_not_logged_in">4</a></li> + <li><a href="/ratings/add/20/52458/5/" title="amazing :-o" class="five-stars_not_logged_in">5</a></li> + </ul> + <span class="numratings">(116)</span> + </div> +</div> + +<div id="single_sample_player"> + <div class="player large"> + <div class="metadata"> + + + <a class="mp3_file" href="/data/previews/52/52458_631427-lq.mp3" + + title="mp3 file">sword-01.wav - mp3 version</a> + + <a class="ogg_file" href="/data/previews/52/52458_631427-lq.ogg" + + title="ogg file">sword-01.wav - ogg version</a> +<a class="waveform" href="/data/displays/52/52458_631427_wave_L.png" + title="waveform file">sword-01.wav - waveform</a> +<a class="spectrum" href="/data/displays/52/52458_631427_spec_L.jpg" + title="spectrogram file">sword-01.wav - spectrogram</a> +<span class="duration" title="duration in seconds">1579.04166667</span> + + + </div> + </div> +</div> + +<div id="single_sample_content"> + <img src="/media/images/40x40_avatar.png" alt="avatar" id="sound_author_avatar" /> + + <div id="sound_author_box"> + <div id="sound_author"><a href="/people/audione/">audione</a></div> + <div id="sound_date">April 29th, 2008</div> + </div> + <div id="sound_description"> + <p>sword blade </p> + </div> + + <ul class="tags"> + + <li><a href="/browse/tags/blade/">blade</a></li> + + <li><a href="/browse/tags/sword/">sword</a></li> + + </ul> + + + <div id="comments_container"> + <h4 id="comments_heading">Comments</h4> + <a name="comments"></a> + <ul id="comments"> + + <li> + <img src="/media/images/32x32_avatar.png" alt="avatar" class="comment_avatar" /> + <div class="comment_info"> + <span class="comment_author"><a href="/people/san_lp79/">san_lp79</a></span> + <span class="comment_date">5 months, 3 weeks ago</span> + + + </div> + <p>Excellent!</p> + + <div style="text-align: right; font-size: 10px; margin-bottom: -8px"> + + <!-- do noting --> + + + </div> + + </li> + + <li> + <img src="/media/images/32x32_avatar.png" alt="avatar" class="comment_avatar" /> + <div class="comment_info"> + <span class="comment_author"><a href="/people/Phil_UK_1970/">Phil_UK_1970</a></span> + <span class="comment_date">6 months ago</span> + + + </div> + <p>Really excellent sound. Thanks!</p> + + <div style="text-align: right; font-size: 10px; margin-bottom: -8px"> + + <!-- do noting --> + + + </div> + + </li> + + <li> + <img src="/media/images/32x32_avatar.png" alt="avatar" class="comment_avatar" /> + <div class="comment_info"> + <span class="comment_author"><a href="/people/Zuurix/">Zuurix</a></span> + <span class="comment_date">6 months ago</span> + + + </div> + <p>Ah, great, going to use it in my GameMaker game. </p> + + <div style="text-align: right; font-size: 10px; margin-bottom: -8px"> + + <!-- do noting --> + + + </div> + + </li> + + <li> + <img src="/data/avatars/3276/3276222_S.jpg" alt="avatar" class="comment_avatar" /> + <div class="comment_info"> + <span class="comment_author"><a href="/people/mrtunze/">mrtunze</a></span> + <span class="comment_date">7 months ago</span> + + + </div> + <p>Sweet sword sound you rock.</p> + + <div style="text-align: right; font-size: 10px; margin-bottom: -8px"> + + <!-- do noting --> + + + </div> + + </li> + + <li> + <img src="/media/images/32x32_avatar.png" alt="avatar" class="comment_avatar" /> + <div class="comment_info"> + <span class="comment_author"><a href="/people/sounderseven/">sounderseven</a></span> + <span class="comment_date">1 year, 1 month ago</span> + + + </div> + <p>This is excellent. A lot of different elements for a rich sound. </p> + + <div style="text-align: right; font-size: 10px; margin-bottom: -8px"> + + <!-- do noting --> + + + </div> + + </li> + + </ul> + + <div id="comments_pagination"> + <ul class="pagination"><li class="disabled-previous-next">previous</li><li class="next-page"><a href="/people/audione/sounds/52458/?page=2#comment" title="Next Page">next</a></li><li class="current-page">1</li><li class="other-page"><a href="/people/audione/sounds/52458/?page=2#comment" title="Page 2">2</a></li><li class="other-page"><a href="/people/audione/sounds/52458/?page=3#comment" title="Page 3">3</a></li><li class="other-page"><a href="/people/audione/sounds/52458/?page=4#comment" title="Page 4">4</a></li><p class="number_of_results"> + +  |  16 comments + + </p></ul> + + + </div> + </div> <!-- comments_container --> + + + <center><p>Please <a href="/home/login/?next=/people/audione/sounds/52458/">log in</a> to comment</center> + +</div> <!-- single_sample_content --> + +<div id="single_sample_sidebar"> + <div id="download"> + + + <a href="/people/audione/sounds/52458/download/52458__audione__sword-01.wav" id="download_login_button" title="login to download"></a> + + + <div id="download_text"><a href="/people/audione/sounds/52458/downloaders/">Downloaded<br /><b>11041</b> times</a></div> + <br style="clear: both;"> + </div> + + + + <div id="sound_license"> + <img src="/media/images/creative_commons.png" with="95" height="23" alt="Creative Commons" /> + <a href="http://creativecommons.org/publicdomain/zero/1.0/">This work is licensed under the Creative Commons 0 License.</a> + </div> + + <dl id="sound_information_box"> + <dt><a href="/help/soundinfo/type/#wav"><img src="/media/images/info.png" width="12" height="12" /></a>Type</dt><dd>Wave (.wav)</dd> + <dt><a href="/help/soundinfo/duration/"><img src="/media/images/info.png" width="12" height="12" /></a>Duration</dt><dd>00:01:579</dd> + <dt><a href="/help/soundinfo/filesize/"><img src="/media/images/info.png" width="12" height="12" /></a>Filesize</dt><dd>444.1 KB</dd> + + + <dt><a href="/help/soundinfo/samplerate/"><img src="/media/images/info_red.png" width="12" height="12" /></a>Samplerate</dt><dd>48000.0 Hz</dd> + <dt><a href="/help/soundinfo/bitdepth/"><img src="/media/images/info_red.png" width="12" height="12" /></a>Bitdepth</dt><dd>24 bit</dd> + + <dt><a href="/help/soundinfo/channels/"><img src="/media/images/info.png" width="12" height="12" /></a>Channels</dt><dd>Stereo</dd> + </dl> + + <ul id="sound_links" style="padding-left: 23px"> + + + + + + <li><a id="similar_sounds_link" rel="nofollow" class="icon" href="/people/audione/sounds/52458/similar/">Similar sounds</a></li> + + + + + + + + + + + + + + </ul> + + <div id="flag_sound"> + Sound illegal or offensive? <a href="/people/audione/sounds/52458/flag/" title="flag this sound as ilegal, offensive or other...">Flag it!</a> + </div> + + <div id="share_links"> + <div id="social_links"> + <div class="social_link" style="width: 50px; overflow: hidden;"><div id="fb-root" style="float: left;"></div><script src="https://connect.facebook.net/en_US/all.js#xfbml=1"></script><fb:like href="http://www.freesound.org/people/audione/sounds/52458/" send="false" layout="button_count" width="55" show_faces="false" font="arial"></fb:like></div> + <a href="https://twitter.com/share" class="twitter-share-button" data-count="none">Tweet</a><script type="text/javascript" src="https://platform.twitter.com/widgets.js"></script> + <span id="tumblr_button_abc123" class="social_link"></span> + <div class="social_link"> + <g:plusone size="medium" count="false" href="http://www.freesound.org/people/audione/sounds/52458/"></g:plusone> + </div> + + <p id="show_button" onclick="$('#embed_links').toggle(500);">embed</p> + <br style="clear: both;" /> + </div> + <div id="embed_links"> + <div id="embed_large" class="embed_link"> + <p>920 x 245</p> + <div class="embed_example embed_large" onclick="selectEmbedSize('large');"></div> + </div> + <div id="embed_medium" class="embed_link"> + <p>481 x 86</p> + <div class="embed_example embed_medium" onclick="selectEmbedSize('medium');"></div> + </div> + <div id="embed_small" class="embed_link"> + <p>375 x 30</p> + <div class="embed_example embed_small" onclick="selectEmbedSize('small');"></div> + </div> + + <textarea id="embed_code" rows="4"></textarea> + + <script type="text/javascript"> + + var tumblr_link_url = "http://www.freesound.org/people/audione/sounds/52458/"; + var tumblr_link_name = "audione - sword-01.wav"; + var tumblr_link_description = "http://www.freesound.org"; + + function selectEmbedSize(size) { + $('.embed_example').removeClass('embed_active'); + $('.embed_example.embed_'+size).addClass('embed_active'); + var sizes; + if(size == 'large') sizes = [920, 245]; + if(size == 'medium') sizes = [481, 86]; + if(size == 'small') sizes = [375, 30]; + var embed_text = '<iframe frameborder="0" scrolling="no" src="http://www.freesound.org/embed/sound/iframe/52458/simple/SIZE/" width="WIDTH" height="HEIGHT"></iframe>'; + embed_text = embed_text.replace('SIZE', size); + embed_text = embed_text.replace('WIDTH', sizes[0]); + embed_text = embed_text.replace('HEIGHT', sizes[1]); + $('#embed_code').text(embed_text); + } + + (function() { + var po = document.createElement('script'); po.type = 'text/javascript'; po.async = true; + po.src = 'https://apis.google.com/js/plusone.js'; + var s = document.getElementsByTagName('script')[0]; s.parentNode.insertBefore(po, s); + })(); + </script> + </div> + <!-- + + <div id="share_content" style="display: none;"> + <p>bla + </div> --> + </div> + + + +</div> <!-- single_sample_sidebar --> + + +<script type="text/javascript"> + var tumblr_button = document.createElement("a"); + tumblr_button.setAttribute("href", "https://www.tumblr.com/share/link?url=" + encodeURIComponent(tumblr_link_url) + "&name=" + encodeURIComponent(tumblr_link_name) + "&description=" + encodeURIComponent(tumblr_link_description)); + tumblr_button.setAttribute("title", "Share on Tumblr"); + tumblr_button.setAttribute("style", "display:inline-block; text-indent:-9999px; overflow:hidden; width:61px; height:20px; background:url('https://platform.tumblr.com/v1/share_2.png') top left no-repeat transparent;"); + tumblr_button.innerHTML = "Share on Tumblr"; + document.getElementById("tumblr_button_abc123").appendChild(tumblr_button); +</script> + + +</div> <!-- END of Content area --> + +<br class="clear" /> + + + + </div> + </div> + + <div id="footer_wrapper"> + <div id="footer"> + <div id="cookie-bar"> + <div style="float:left"> + <p> + We use cookies to ensure you get the best experience on our website. By browsing our site you agree to our use of cookies. + <br> + For more information check out our <a href="/help/cookies_policy">cookies policy</a>. + </p> + </div> + <div style="float:right; margin-top:10px;margin-right:10px;"> + <button id="cookie-accept">Ok</button> + </div> + <br class="clear"> + + </div> + <div id="footer_links"> + <a href="/help/developers/">Developers</a> <a href="http://blog.freesound.org/">Blog</a> <a href="/help/about/">About</a> <a href="/contact/">Contact</a> <a href="/help/tos_web/">Terms of use</a> + </div> + <div id="footer_credits"> + Design by <a href="http://www.pixelshell.com">Pixelshell</a>. Code by <a href="http://www.mtg.upf.edu/">MTG (UPF)</a>. Some Rights Reserved. + </div> + </div> + </div> + + </body> + +</html>